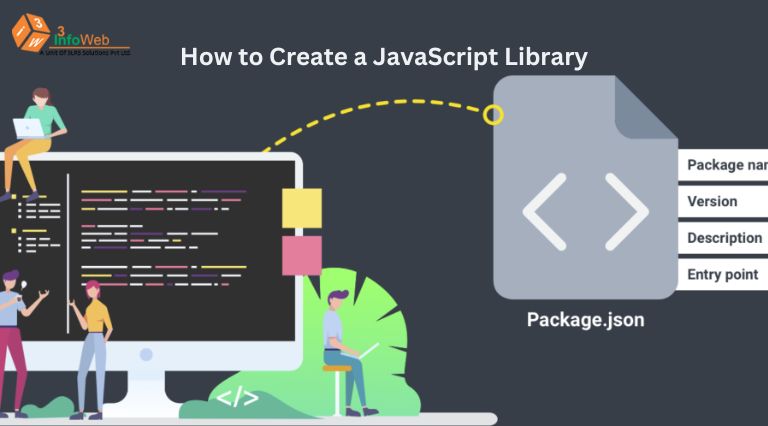
How to Create a JavaScript Library, Full Guidance for Beginners
JavaScript libraries are powerful tools that simplify the development process, enhance code reusability, and promote efficient coding practices. Creating your own JavaScript library can be a rewarding experience, allowing you to contribute to the developer community and streamline your projects. In this guide, we’ll walk you through the essential steps to create a JavaScript library, catering specifically to beginners.
What is JavaScript?
JavaScript is like the Swiss Army knife of the web development world, and what makes it truly unique can be summed up in a few simple points:
Client-Side Scripting
JavaScript is like the magic wand for websites. It’s a special language that works behind the scenes in your web browser. Imagine it as a wizard that can change how a webpage looks and behaves. It’s the reason web pages can do cool things like pop-ups, animations, and dynamic updates without needing to reload. So, when you see a webpage doing more than just sitting there, chances are JavaScript is the secret sauce making it all happen
Interactivity and Responsiveness
JavaScript is like the superhero of the web world. It helps developers add awesome things to websites, like making sure you fill out forms correctly, creating cool image slideshows, and even building interactive maps that you can explore. It’s like giving websites a brain that instantly reacts when you click or type something, making your online experience way more fun and exciting
ECMAScript Specification
JavaScript has a rule book called ECMAScript that tells it how to do cool tricks. Think of it like a recipe that helps JavaScript work its magic. Whether it’s in a web browser or a special place for code called Node.js, they all follow this same recipe. People sometimes call JavaScript by the name ECMAScript, kind of like how a nickname is used for a best friend—it’s just another way to talk about the same clever language
Multi-Paradigm
JavaScript is like a versatile toolbox for developers. It’s not stuck doing just one thing—it can play the role of an actor in a play or a chef in the kitchen. In programming terms, it can be both a smooth talker (object-oriented) and a problem-solving wizard (functional). Depending on what needs to be done, developers get to pick the best approach from their toolkit to make their projects shine
Asynchronous Programming
JavaScript is like a multitasking superhero in the programming world. It doesn’t get stuck waiting around—it can do one thing while keeping an eye on another. For example, when fetching information from a faraway server, JavaScript doesn’t just twiddle its thumbs. It uses special tricks like callbacks, Promises, and the async/await magic words to keep things moving smoothly. It’s like having a superhero that can juggle tasks effortlessly, making sure everything gets done without a hitch
Cross-Platform Development
JavaScript was like a garden tool specifically made for making websites more awesome. But then, something amazing happened! A clever idea called Node.js came along and said, “Hey, let’s use JavaScript for more than just making web pages look cool.” So now, thanks to Node.js, JavaScript isn’t just a decorator for websites—it’s like a superhero that can handle everything from making the front of a website shine to managing all the behind-the-scenes stuff on the server. It’s like JavaScript put on a cape and became a full-stack superhero for building all sorts of incredible online things
Also Read: Top10 Best Free Online Video Editor, A-to-Z Guide Full Information For Beginners
Libraries and Frameworks
JavaScript has a vast ecosystem of libraries and frameworks that simplify and accelerate development. Libraries like jQuery and frameworks like React, Angular, and Vue.js provide tools and structures for building scalable and maintainable applications.
Modern JavaScript (ES6+)
Imagine JavaScript leveling up to become even cooler! That’s what happened with ECMAScript 6 (or ES6), which is like the superhero name for JavaScript in the year 2015. It brought in fancy new tools like arrow functions (super quick and efficient), classes (like a blueprint for creating things), and template literals (fancy ways to write text). And guess what? JavaScript didn’t stop there. It kept upgrading in later versions, getting even more awesome features. So, it’s like JavaScript went to a tech makeover and now has all these cool, modern tricks up its sleeve
Community and Resources
JavaScript is like a popular hangout spot for tech enthusiasts. Picture it as a bustling town where developers gather to share their knowledge. There’s a treasure trove of guides, step-by-step lessons, and friendly chats happening all the time. Whether you’re just starting or you’ve been coding for a while, it’s like having a big, welcoming community that’s always ready to help you on your JavaScript journey. It’s like a digital neighborhood where everyone’s invited to learn and grow together
Tooling
JavaScript is like a superhero’s toolkit for building amazing websites. But here’s the secret sauce: it doesn’t work alone. It has these cool sidekicks—think of them like trusty companions on a quest. There’s npm, the magical backpack that holds all the helpful stuff. Then there’s Webpack, the expert builder that puts everything in the right place. And don’t forget Jest, the superhero tester who makes sure everything works perfectly.
So, learning JavaScript isn’t just about the language itself; it’s like joining a superhero squad with these powerful helpers. It’s not just coding; it’s an adventure in a world where websites come to life, and you get to be the hero who makes it happen
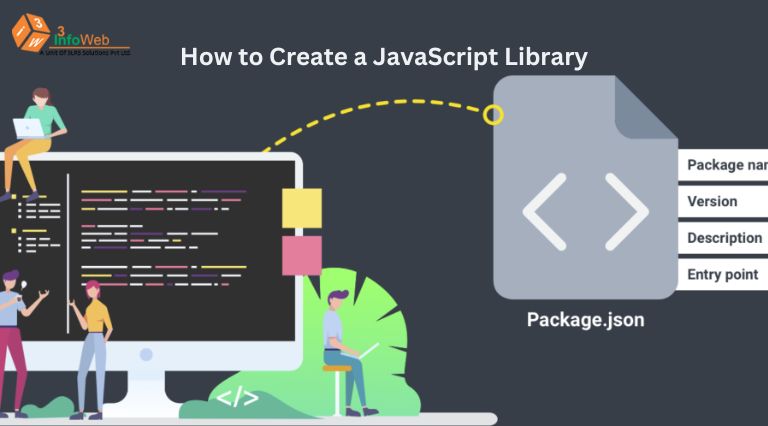
What is JavaScript Library?
JavaScript libraries are like ready-made toolboxes for developers. Imagine you’re building something cool with LEGO bricks. Instead of making every piece from scratch, you grab a box filled with pre-designed, handy parts. That’s what a JavaScript library does—it’s a collection of shortcuts and tricks created by smart developers.
So, instead of spending ages writing code for common tasks, you can grab a piece from the library. It’s like having a superhero sidekick that helps you do things faster and with less effort. Your job? Focus on the fun and creative parts of building, while the library takes care of the nitty-gritty details. It’s coding made easy and awesome
Also Read: What are the Best AI and Marketing Automation Tools?
Here are some key points about JavaScript libraries:
1. No Reinventing the Wheel: Libraries have ready-made code for common tasks. You don’t need to start from scratch every time you want to do something cool.
2. Time Saver: Think of libraries as time-traveling assistants. They bring in tested and optimized code, so you don’t spend forever writing everything yourself.
3. Easy Peasy: Libraries make tricky stuff simple. They take complex jobs and turn them into easy-to-use tools, like turning a spaceship dashboard into a single button.
4. Browser Peace: Ever worry if your code will behave differently in different browsers? Libraries often handle that for you, ensuring your creation works smoothly everywhere.
5. Team Spirit: Libraries are like community potlucks. They’re made and looked after by a bunch of friendly developers. That means they’re always getting better, and there’s a whole gang ready to help you out if you need it.
Examples of Super Tools (JavaScript Libraries):
- jQuery: The superhero for handling webpage tricks like magic.
- React, Angular, Vue.js: Imagine them as the architects making sure your website looks and behaves just the way you want.
- Lodash: The wise helper that brings extra powers for dealing with data.
- Chart.js: The artist that turns boring numbers into stunning charts and graphs.
Also Read: How to Use Instagram Analytics, Unleashing the Power of Instagram Analytics
How to Create a JavaScript Library
Creating a JavaScript library involves several steps. Here’s a more detailed guide to help you through the process:
Purpose: First, figure out what flavor your cake (library) should have. What problem does it solve or what special ability does it give to developers?
API Planning: Think about how you want people to enjoy your cake. Design a menu (API) that’s easy to understand. What functions or features will they interact with?
Project Structure: Imagine your kitchen shelves. Organize your ingredients (code) neatly in different sections like source code, testing, documentation, and the final cake (distribution files).
Initialize Project: Open your recipe book (version control system) and create a shopping list (package.json). It’s like a magical book that contains everything about your cake, from ingredients to cooking instructions.
Write the Code: Time to mix the ingredients! Write the actual code, breaking it into bite-sized pieces. Follow the rules of baking (coding style and structure).
Testing: Before serving the cake, make sure it’s delicious! Write tests to check if everything tastes right. Tools like a magical taste-tester (testing framework) help.
Documentation: Imagine a cookbook with clear instructions. Document your recipe (code) using tools like JSDoc, so others can recreate the magic.
Versioning: Sometimes you tweak the recipe. Follow a cookbook versioning system. If you add a secret ingredient, change the version number.
Build Process: In baking, you prep the cake before baking. Similarly, if needed, bundle and transform your code using magical tools like a baking oven (build tools).
Publishing: Ready to share your cake with the world? Publish it to a magical cookbook store (npm for JavaScript libraries). Open an account, log in, and share the recipe (publish).
Licensing: Imagine putting a label on your cake with rules. Choose a license that says how others can enjoy, share, and tweak your recipe.
Community and Support: Open a café (GitHub repository) where people can talk about your cake. Encourage them to share their versions, ask questions, and make your cake even more amazing.
Also Read: Top 10 Essential Skills Every Digital Marketer Needs to Know
FAQs On How to Create a JavaScript Library, Full Guidance for Beginners
Q1: What is a JavaScript library, and why would I want to create one?
Ans: A JavaScript library is a collection of pre-written, reusable code that helps developers perform common tasks without starting from scratch. Creating a library is like sharing your coding superpowers with others, making it easier for them to build amazing things.
Q2: Do I need to be an expert in JavaScript to create a library?
Ans: Not necessarily. While some coding knowledge is helpful, the process of creating a library is a fantastic way to learn and improve your skills. This guide is designed to help beginners navigate the steps.
Q3: How do I decide the purpose of my library?
Ans: Think about common problems or tasks in web development that could be made easier. Your library could solve a specific problem, like handling data or creating visual elements, or provide a handy set of tools for developers.
Q4: What’s an API, and why is it important in library creation?
Ans: An API (Application Programming Interface) is like a menu that defines how users interact with your library. It’s crucial to plan a clean and intuitive API to make using your library straightforward and enjoyable.
Q5: What’s the best way to structure my project?
Ans: Imagine your project as a house. Create separate rooms (directories) for source code, testing, documentation, and distribution files. This organization makes it easier for you and others to navigate.
Q6: How do I start the project and set it up for version control?
Ans: Initiate your project with a version control system like Git. Create a “package.json” file to store metadata, dependencies, and scripts. Use the “npm init” command to kick things off.
Q7: Can you explain more about writing the actual code?
Ans: Break down your code into smaller, manageable parts. Follow coding best practices, and consider modular components. This is where you bring your library’s purpose to life.
Q8: Why is testing important, and how do I do it?
Ans: Testing ensures your library works as expected. Use testing frameworks like Mocha or Jest. Write tests that cover different scenarios. This helps catch bugs early and keeps your library reliable.
Q9: What should I consider when documenting my code?
Ans: Think of documentation as a recipe book. Use tools like JSDoc to explain each function and provide examples. Good documentation is key for users to understand and use your library effectively.
Q10: How do I handle versioning and why is it necessary?
Ans: Versioning follows semantic versioning (SemVer) principles. It helps users know if there are changes and if they need to update their code. Update the version number in your “package.json” file with each release.